VB Lecture Notes - For Loops
Objective #1: Use For loops.
- A For loop allows you to repeat a section of code many times over and over again. In the following For loop, five message boxes will appear one after the other
For J = 1 To 5
MessageBox.Show("Hello World")
Next
The line of code MessageBox.Show("Hello World") is considered to be inside the body of the loop since it is between the For and Next statements. It is good style to indent the lines of code that are in the body of the loop.
In the example above, the variable J is considered to be the loop variable. You can use name for the loop variable but usually it does not make sense to name the loop variable something like numDonuts or totalCost. In fact, the variable name J is very often used as a For loop variable by professional programmers.
- Each pass of the loop is called an iteration. The loop above iterates 5 times. On the first pass through the loop J is equal to 1. Then J increments to 2 and the loop iterates a second time. Then J increments to 3 and the loop iterates again. Then J increments to 4 and the loop iterates again. Finally J increments to 5 and the loop iterates yet again. Finally J increments to 6 and the loop stops because J is greater than the upper boundary of 5. In the example above, each time the loop iterates a message box pops up with "Hello World". Loop variables like J in this type of For loop automatically increment by one each time around the loop.
- A For loop is called
a definite loop (or determinate loop) because the programmer knows exactly how many times the code will be repeated. If you know exactly how many iterations are necessary for a certain task, you should use a For loop rather than another kind of loop that you will study later called
a While loop.
- Here's another example of a For loop:
For J = 1 To 5
MessageBox.Show("Hello World")
Next
In this example, J begins with the value of 1. Each time the loop iterates the J increases
by one. So J will be equal to 2 the second time around the loop and so on. When J is equal to 5 the loop will
iterate one last time. Overall, the loop will iterate exactly 5 times. Each time the loop iterates the computer will display a message box.
- The loop variable can change by amounts other than 1 on each iteration. The amount that the loop variable changes is called the loop's step size. The default step size for
Visual Basic For loops is 1. However, you can type the keyword Step followed
by any other value to change the step size.
Examples:
For J = 2 To 10 Step 2 ' 5 iterations since J becomes 2, 4, 6, 8, 10 (and then 12)
MessageBox.Show(J) ' The value of J prints instead of the phrase "Hello World"
Next
For J = 2 To 11 Step 2 ' 5 iterations even though J doesn't "land" exactly on 11
MessageBox.Show("Hello World")
Next
For J = 10 To 50 Step 10 ' 5 iterations since J becomes 10, 20, 30, 40, 50 (and then 60)
MessageBox.Show("Hello World")
Next
For J = 5 To 1 Step -1 ' 5 iterations since J becomes 5, 4, 3, 2, 1 (and then 0)
MessageBox.Show("Hello World")
Next
- If you use a step size of 1, do not type out the "Step 1" part of the For statement. You will lose points
on programs that include this unnecessary code. It is considered to be acceptable style to use loop variables that consist of a name with one letter such as J.
- If a For loop has a Step value of 1 as in
For J = low To high
iterations = iterations + 1
Next
then the number of loop iterations can easily be calculated with the formula
number of loop iterations = High - Low + 1
Be careful of the fencepost error which results from not adding 1 in this formula. The fencepost error is dangerous since it causes computer programmers to underestimate the overall number
of loop iterations by one. If you built a fence with 4 fencerails then you need 5 fenceposts. That is, you need one more fencepost than fencerails. The fencepost error is also known as an off-by-one-bug
(OBOB).
- An Exit For statement can be used to immediately end a For loop. In the following example, only 3 message boxes appear since the the Exit For statement causes the loop to immediately end when J is 3. We will not be studying If End If statements until later in the school year.
For J = 1 To 10
MessageBox.Show("Hello World")
If (J = 3) Then
Exit For
End If
Next
- Be careful not to create infinite loops that iterate forever. The following For loop may iterate forever
For J = 1 To 10 Step -1
MessageBox.Show("Hello World")
Next
Objective #2: Trace For loops.
- It is important to be able to trace a loop. Tracing a loop means that you analyze it and execute it in your mind to predict what happens and to count how many iterations it makes. When you trace a loop you must keep track of the values stored in any variables. You must make a column for each variable involved in the For loop and show the corresponding values stored in those variables as the loop iterates. I prefer that you cross out the intermediate values and then circle the final value stored in each variable.
Example #1:
Dim sum
As Integer = 0
For J = 1 To 5
sum = sum + 1
Next |
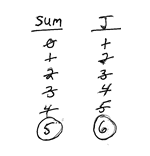 |
The Step amount for the loop variable J is considered to be 1. So the loop variable J increments by 1 on each iteration and the final value stored in J when the loop is completely finished is 6. Take note that J ends up going over the upper boundary of 5 since that is how the loop knows to end. The final value stored in sum is 5 since the counter statement sum = sum + 1 increases sum also by one on each iteration.
You could think of the loop above as a simulation for the following scenario. Pretend that someone is giving you a $1 each hour starting at 1 p.m. and ending at 5 p.m. You can probably figure out mentally that you will end up with a sum of $5. The loop above models that situation and the variable sum will be equal to 5 by the end of the algorithm.
Example #2:
Dim sum
As Integer = 0
For J = 2 To 10 Step 2
sum = sum + 1
Next |
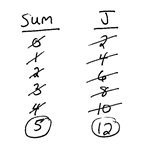 |
The final value stored in J when the loop is completely finished is 6. Take note that J goes up by two's in this example (due to the Step amount of 2) and it ends up going over the upper boundary of 10 since that is how the loop knows to end. Again in this example the final value stored in sum is 5.
Example #3:
Dim sum
As Integer = 0
For J = -3 To 0
sum += 1
Next |
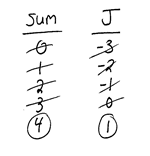 |
The final value stored in J when the loop is completely finished is 1. Take note that J ends up going over the upper boundary of 0 since that is how the loop knows to end. The final value stored in sum is 4. Also notice in this example that the counter statement sum += 1 is used instead of the equivalent sum = sum + 1.
Example #4:
Dim sum
As Integer = 0
For J = 1 To 5
sum = sum + J
Next |
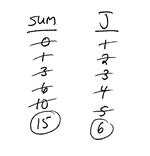 |
The final value stored in J when the loop is completely finished is 6. The final value stored in sum is 15. Since J varies on each iteration, sum goes up by a different amount on each loop iteration. You are not adding a fixed amount to sum like 1 or 2.
Example #5:
Dim sum
As Integer = 0
For J = 1 To 5
sum += 1
Next |
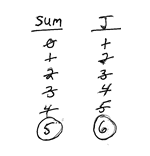 |
This example is the same as Example #1 except that the counter statement sum = sum + 1 is retyped as sum += 1 by making use of the += compound operator.
Objective #3: Trace double-nested For loops.
- You will not be tested on the following material however it is worthwhile to understand double-nested loops.
- A nested For loop is a For loop
inside of another For loop.
The two loops together are called double-nested For loops. Example:
Dim R As Integer = 0
Dim C As Integer = 0
For R = 1 To 3
For C = 1 To 2
lblOutput.Text = lblOutput.Text + R * C
Next
lblOutput.Text = lblOutput.Text + vbCrLF
Next
Notice how the nested For loop is indented inside of the outer For loop for good style. Also notice how blank lines are placed around loops for good style and increased
readability.
We will trace this example in class.